User touch input
You are to first successfully copy, paste, build and run the app before doing anything else. The instructions are at the beginning of the source code below.
1) Single-touch
/*
Simple code to get the user single-touch input
and draw a rectangle where the screen is touched.
It is assumed that you already followed the
content of the page on 'Simple draw pixels'.
To build and run the android app, first
follow the steps to 'Get everything ready'.
Then inside the project folder and inside src/com/example/,
replace the entire code in MainActivity.java file with this code.
Use at your own risk!
*/
package com.example.myfirstapp;
import android.os.Bundle;
import android.app.Activity;
import android.content.Context;
import android.graphics.Canvas;
import android.view.MotionEvent;
import android.view.View;
public class MainActivity extends Activity
{
/** Called when the activity is first created. */
@Override
public void onCreate (Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
//setContentView(R.layout.main); // this is the default view but which is replaced.
setContentView(new MyView(this)); // the activity will have only one view 'MyView'.
}
private class MyView extends View // custom view so to override onDraw()
{
int width, height;
int[] pixels_colours;
boolean firstTime = true;
public MyView(Context context) // class constructor that must be present
{ super(context); }
@Override // here is the purpose of extending the View class
protected void onDraw(Canvas canvas)
{
if(firstTime)
{
firstTime = false;
// Get width and height of the View. These will be for the
// full area taken by the activity since it has only one view.
width = getWidth();
height = getHeight();
// array to store the colours for all the pixels
pixels_colours = new int[height*width];
for(int y=0; y<height; y++)
for(int x=0; x<width; x++)
pixels_colours[y*width+x] = (x + y<<16); // any colour
}
// draw to screen of device
canvas.drawBitmap (pixels_colours, 0, width, 0, 0, width, height, false, null);
}
void myDrawRectangle (int x, int y, int colour)
{
for(int i=x-4; i<x+4; i++)
for(int j=y-4; j<y+4; j++)
if(i>=0 && i<width && j>=0 && j<height)
pixels_colours[j*width+i] = colour;
}
@Override
public boolean onTouchEvent (MotionEvent event)
{
int x = (int)event.getX(); // get the (x,y) position
int y = (int)event.getY(); // relative to the view
switch (event.getAction())
{
case MotionEvent.ACTION_DOWN: // on a press,
myDrawRectangle(x, y, 0x000000FF); // draw a blue rectangle
break;
case MotionEvent.ACTION_MOVE: // on a move,
myDrawRectangle(x, y, 0x0000FF00); // draw a green rectangle
break;
case MotionEvent.ACTION_UP: // on a release,
myDrawRectangle(x, y, 0x00FF0000); // draw a red rectangle
break;
case MotionEvent.ACTION_CANCEL: // on a cancellation,
myDrawRectangle(x, y, 0x00000000); // draw a black rectangle
break;
default: return false; // may not be needed
}
invalidate(); // schedule a refresh of the View
return true;
// more at http://developer.android.com/reference/android/view/MotionEvent.html
}
}
}
Result is
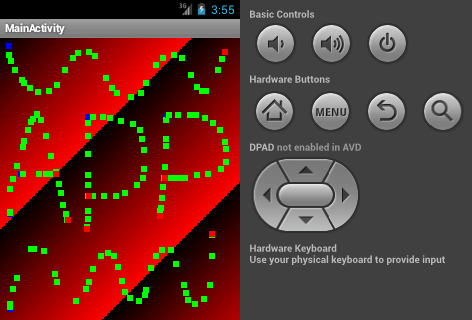
2) Multi-touch
Some devices can report multiple movement traces at the same time. This is described as multi-touch. To implement multi-touch, replace the entire method onTouchEvent() of the code above with the modified version below.
/* Read the code, let it speak for itself! */
@Override
public boolean onTouchEvent (MotionEvent event)
{
int x, y, index, count;
switch (event.getAction())
{
case MotionEvent.ACTION_DOWN:
case MotionEvent.ACTION_POINTER_DOWN:
case MotionEvent.ACTION_UP:
case MotionEvent.ACTION_POINTER_UP:
case MotionEvent.ACTION_MOVE:
count = event.getPointerCount();
for(index=0; index < count; index++)
{
x = (int)event.getX(index);
y = (int)event.getY(index);
// draw the rectangle with a colour
// that depends on the pointer index
myDrawRectangle(x, y, 0xFF<<(8*index));
}
break;
case MotionEvent.ACTION_CANCEL:
return false;
default: return false; // may not be needed
}
invalidate(); // schedule a refresh of the View
return true;
}
Basically, the method/function of interest here is onTouchEvent(), and the class of interest here is MotionEvent.
Contact us to report broken links and errors.